Set Up ESLint and Prettier in a React TypeScript App 2022
Linting and Formatting using ESLint & Prettier
Once you start using TypeScript with React, there is no going back. You would simply fall in love with the ease it provides. But to establish a standard for your team on how to format the codebase, it's important to have the linter and formatter in place to ensure that the code is consistent irrespective of the developer writing the code. ESLint and Prettier are the favorites of the developer community when it comes to linting and formatting the code. If you are looking to set up ESLint and Prettier for JavaScript-based React applications, I would suggest you have a look at my article for the same: Set Up ESLint and Prettier in a React App with Absolute Imports (2022)
Let’s look at how to set up ESLint and Prettier for a React TypeScript project. Do note that I’m using Vite for scaffolding the react project for the course of this article.
1. Install Dependencies
To set up ESLint and Prettier, we would be required to add a bunch of dependencies in our application like eslint, eslint-config-airbnb, prettier using the command given right below.
yarn add -D @typescript-eslint/eslint-plugin@^5.13.0 @typescript-eslint/parser@^5.0.0 eslint@^8.24.0 eslint-config-airbnb@^19.0.4 eslint-config-airbnb-typescript@^17.0.0 eslint-config-prettier@^8.5.0 eslint-import-resolver-typescript@^3.5.1 eslint-plugin-import@^2.26.0 eslint-plugin-jsx-a11y@^6.6.1 eslint-plugin-prettier@^4.2.1 eslint-plugin-react@^7.31.8 eslint-plugin-react-hooks@^4.6.0 prettier@^2.7.1
The exact version to be used for a particular dependency is mentioned as they are dependent on each other to function correctly but if you want to play around a bit with the latest versions, you may use this command to install the latest versions:
yarn add -D @typescript-eslint/eslint-plugin @typescript-eslint/parser eslint eslint-config-airbnb eslint-config-airbnb-typescript eslint-config-prettier eslint-import-resolver-typescript eslint-plugin-import eslint-plugin-jsx-a11y eslint-plugin-prettier eslint-plugin-react eslint-plugin-react-hooks prettier
2. Add ESLint Config file
The ESLint config can either be generated via the command line using the command eslint --init and answering a few questions regarding the code conventions to be followed or using some existing configuration like the one given below. This configuration already solves the problem of conflicting rules between prettier and ESLint for quotes and indentation. To use this configuration, you would be required to create a new file .eslintrc.json at the root of your project.
{
"env": {
"browser": true,
"es6": true
},
"extends": [
"eslint:recommended",
"airbnb/hooks",
"airbnb-typescript",
"plugin:react/recommended",
"plugin:react/jsx-runtime",
"plugin:@typescript-eslint/recommended",
"plugin:@typescript-eslint/recommended-requiring-type-checking",
"plugin:prettier/recommended",
"plugin:import/recommended"
],
// Specifying Parser
"parser": "@typescript-eslint/parser",
"parserOptions": {
"ecmaFeatures": {
"jsx": true
},
"ecmaVersion": "latest",
"sourceType": "module",
"tsconfigRootDir": ".",
"project": [
"./tsconfig.json"
]
},
// Configuring third-party plugins
"plugins": [
"react",
"@typescript-eslint"
],
// Resolve imports
"settings": {
"import/resolver": {
"typescript": {
"project": "./tsconfig.json"
}
},
"react": {
"version": "18.x"
}
},
"rules": {
"linebreak-style": "off",
// Configure prettier
"prettier/prettier": [
"error",
{
"printWidth": 80,
"endOfLine": "lf",
"singleQuote": true,
"tabWidth": 2,
"indentStyle": "space",
"useTabs": true,
"trailingComma": "es5"
}
],
// Disallow the `any` type.
"@typescript-eslint/no-explicit-any": "warn",
"@typescript-eslint/ban-types": [
"error",
{
"extendDefaults": true,
"types": {
"{}": false
}
}
],
"react-hooks/exhaustive-deps": "off",
// Enforce the use of the shorthand syntax.
"object-shorthand": "error",
"no-console": "warn"
}
}
Feel free to play around with the rules defined above or extend some of the other configurations like eslint-config-google
to figure out what’s best suited for your project.
3. Update Scripts
Now to test the linting commands let’s update the scripts in our package.json file by adding the commands given below:
"lint": "tsc --noEmit && eslint src/**/*.ts{,x} --cache --max-warnings=0",
"lint:fix": "eslint src/**/*.ts{,x} --fix"
The --max-warnings
flag helps to ensure that the developed code is lint properly before committing the code using the pre-commit checks without any warning while the --fix flag auto-fixable errors/warnings. Once this is done, we are ready to test the ESLint configuration by running the following command which would scan all the JavaScript files in the project.
yarn run lint
4. Update Settings for VSCode
Optionally, we can update the VSCode Configuration such that it displays the linting and formatting errors and fix the auto-fixable ones on saving the file. To achieve this, we would be required to install a few extensions: ESLint and Prettier. I also recommend installing Error Lens which helps in highlighting the errors/warnings in the file itself while writing the code. Once this is done, we would require to add a new .vscode/settings.json file to the root of the project as shown below:
{
"editor.formatOnSave": true,
"editor.defaultFormatter": "esbenp.prettier-vscode",
"prettier.trailingComma": "es5",
"prettier.requireConfig": false,
"prettier.singleQuote": true,
"prettier.useTabs": true,
"prettier.tabWidth": 2,
"prettier.printWidth": 80,
"editor.tabSize": 2,
"editor.codeActionsOnSave": [
"source.formatDocument",
"source.fixAll.eslint"
],
}
5. Add .eslintignore File
Once the setup is complete, ESLint and Prettier might try to lint the build files as well in the dist/ folder. To avoid it, we can create a .eslintignore file and add dist to it. It informs ESLint to skip looking into the dist folder.
Conclusion
Once all of this configuration is done, ESLint and Prettier should be up and running for linting and formatting your project as shown in the attached screenshot:
As always, here is a link to the complete setup on Github.
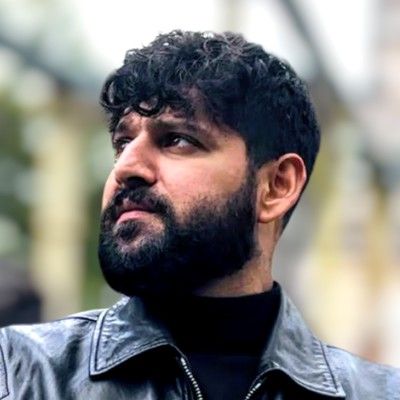